After spending most of my life learning and trying to master C++, I have recently decided to give Rust a try. I only have one project, but I really enjoy the language thus far.
fn main() {
let n = 5;
if n < 0 {
print!("{} is negative", n);
} else if n > 0 {
print!("{} is positive", n);
} else {
print!("{} is zero", n);
}
}
Lucky Numbers
Lucky Numbers are a concept introduced to me by one of my professors Dr. Duane Skaggs. And one of the first Rust projects that I have worked on, but before I get to the rust code, I should explain what lucky numbers are.
Also…
Therefore 11 is a lucky number. In general, a lucky number is any integer which can be expressed as the sum of integers whose reciprocals add to one.
Take a moment to find some lucky numbers on your own. This dropdown contains the first 11
\(1, 4, 9, 10, 11, 16, 18, 20, 22, 24, 25\)These are the first 11 lucky numbers. One of these numbers actually has 3 unique constructions.
Doubly Lucky Numbers
Some numbers have multiple have multiple ways to construct them in a lucky way, take 31 for example:
31 has two constructions, in this case we would say it has a luckiness of 2
Finding Lucky Numbers
I believe that there are many ways to find lucky numbers, however the that I found works by starting with a common denominator and constructing lucky numbers from that number.
For example, with the number 4 as a common denominator, you can find the following fractions which add to 1
These correspond to the lucky numbers of 16 and 10, and it gives their construction by simplifying the fractions.
Rust Console Application
I made a repo with the following 4 functionalities
is_lucky
Prints if a number in a range is lucky
$ luckynums is_lucky 10 25
10: true
11: true
12: false
13: false
14: false
15: false
16: true
17: false
18: true
19: false
20: true
21: false
22: true
23: false
24: true
25: true
luckiness
Prints the luckiness of a number in a given range
$ luckynums luckiness 1 35
1: 1
2: 0
3: 0
4: 1
5: 0
6: 0
7: 0
8: 0
9: 1
10: 1
11: 1
12: 0
13: 0
14: 0
15: 0
16: 1
17: 0
18: 1
19: 0
20: 1
21: 0
22: 3
23: 0
24: 1
25: 1
26: 0
27: 1
28: 1
29: 1
30: 1
31: 2
32: 1
33: 2
34: 1
35: 1
constructions
Prints the constructions for lucky numbers in a given range
$ luckynums constructions 1 35
1: 1
2 [Not Lucky]
3 [Not Lucky]
4: 2*2
5 [Not Lucky]
6 [Not Lucky]
7 [Not Lucky]
8 [Not Lucky]
9: 3*3
10: 4*2 + 2
11: 6 + 3 + 2
12 [Not Lucky]
13 [Not Lucky]
14 [Not Lucky]
15 [Not Lucky]
16: 4*4
17 [Not Lucky]
18: 6*2 + 3*2
19 [Not Lucky]
20: 6*3 + 2
21 [Not Lucky]
22: 8*2 + 4 + 2
10 + 5*2 + 2
12 + 4 + 3*2
23 [Not Lucky]
24: 12 + 6 + 4 + 2
25: 5*5
26 [Not Lucky]
27: 6*4 + 3
28: 8*2 + 4*3
29: 12*2 + 3 + 2
30: 12 + 6 + 4*3
31: 12 + 6*2 + 4 + 3
20 + 5 + 4 + 2
32: 18 + 9 + 3 + 2
33: 9*3 + 3*2
15 + 5*3 + 3
34: 8*4 + 2
35: 12*2 + 4*2 + 3
plot
Outputs a graph to chart.png using the plotters crate
$ luckynums plot 1 50
Results saved to chart.png
Chart.png:
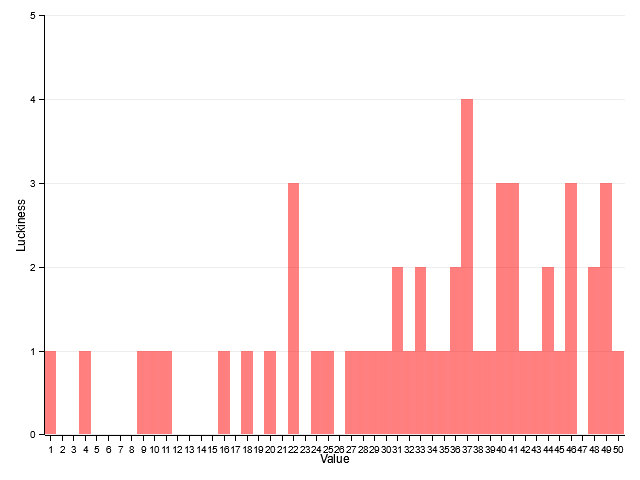